API Map - Circle Area
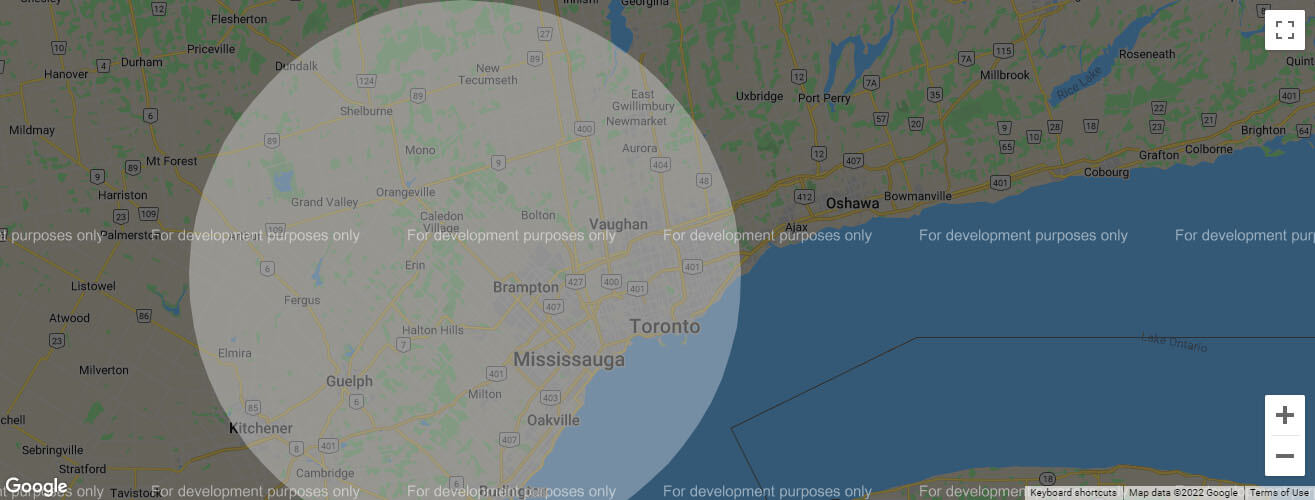
HTML
<!--API Map - Circle Area-->
<div class="map">
<div id="map"></div>
</div>
<script async type="text/javascript">
var citymap = {
serviceArea: {
center: {lat: 43.75, lng: -79.93},
area: 371487
}
};
function initializeMap() {
var w = Math.max(document.documentElement.clientWidth, window.innerWidth || 0);
var isDraggable = w > 480 ? true : false;
var map = new google.maps.Map(document.getElementById('map'), {
scrollwheel: false,
zoom: 9,
center: new google.maps.LatLng(43.771878, -79.453803),
navigationControl: false,
mapTypeControl: false,
scaleControl: false,
draggable: isDraggable
});
for (var city in citymap) {
var cityCircle = new google.maps.Circle({
strokeWeight: 0,
fillColor: '#ffffff',
fillOpacity: 0.32,
map: map,
center: citymap[city].center,
radius: Math.sqrt(citymap[city].area) * 100
});
}
var customMapType = new google.maps.StyledMapType(
[
{
"featureType": "water",
"elementType": "geometry",
"stylers": [
{
"color": "#333739"
}
]
},
{
"featureType": "landscape",
"elementType": "geometry",
"stylers": [
{
"color": "#2ecc71"
}
]
},
{
"featureType": "poi",
"stylers": [
{
"color": "#2ecc71"
},
{
"lightness": -7
}
]
},
{
"featureType": "road.highway",
"elementType": "geometry",
"stylers": [
{
"color": "#2ecc71"
},
{
"lightness": -28
}
]
},
{
"featureType": "road.arterial",
"elementType": "geometry",
"stylers": [
{
"color": "#2ecc71"
},
{
"visibility": "on"
},
{
"lightness": -15
}
]
},
{
"featureType": "road.local",
"elementType": "geometry",
"stylers": [
{
"color": "#2ecc71"
},
{
"lightness": -18
}
]
},
{
"elementType": "labels.text.fill",
"stylers": [
{
"color": "#ffffff"
}
]
},
{
"elementType": "labels.text.stroke",
"stylers": [
{
"visibility": "off"
}
]
},
{
"featureType": "transit",
"elementType": "geometry",
"stylers": [
{
"color": "#2ecc71"
},
{
"lightness": -34
}
]
},
{
"featureType": "administrative",
"elementType": "geometry",
"stylers": [
{
"visibility": "on"
},
{
"color": "#333739"
},
{
"weight": 0.8
}
]
},
{
"featureType": "poi.park",
"stylers": [
{
"color": "#2ecc71"
}
]
},
{
"featureType": "road",
"elementType": "geometry.stroke",
"stylers": [
{
"color": "#333739"
},
{
"weight": 0.3
},
{
"lightness": 10
}
]
}
], {
name: 'Custom Style'
});
var customMapTypeId = 'custom_style';
map.mapTypes.set(customMapTypeId, customMapType);
map.setMapTypeId(customMapTypeId);
}
google.maps.event.addDomListener(window, 'load', initializeMap);
</script>