Map Styles: Snazzy Maps
API Map - Multiple Locations
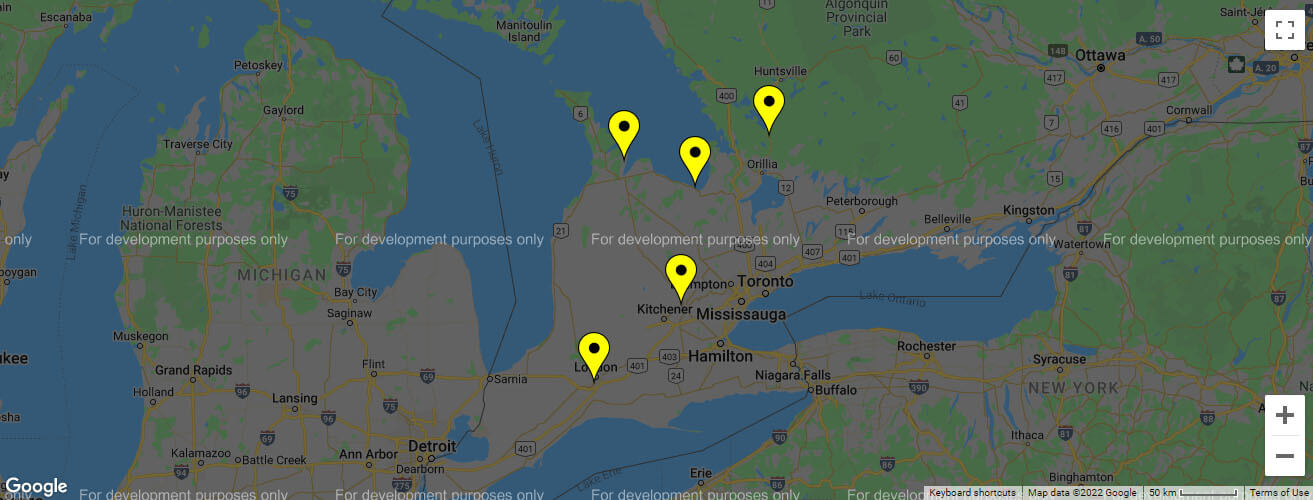
HTML
<!--API Map - Multiple Locations-->
<div class="map">
<div id="map"></div>
</div>
<script type="text/javascript">
var LocationData = [
[43.5489907, -80.3097019, "<div class='marker_info'><p>GUELPH OFFICE</p><p>54 Monarch Rd, Unit-1, Guelph, ON</p><p>Phone: <a href='tel:+1519-826-6969'>+1519-826-6969</a></p></div>"],
[44.6804908, -80.9335211, "<div class='marker_info'><p>OWEN SOUND OFFICE</p><p>319414 Grey Rd 1, Owen Sound, ON</p><p>Phone: <a href='tel:+1519-371-1500'>+1519-371-1500</a></p></div>"],
[42.9214824, -81.2628909, "<div class='marker_info'><p>LONDON, ON OFFICE</p><p>207 Exeter Road, London, ON</p><p>Phone: <a href='tel:+1519-668-1555'>+1519-668-1555</a></p></div>"],
[44.4825997, -80.1573887, "<div class='marker_info'><p>COLLINGWOOD OFFICE</p><p>9485 Beachwood Road, Collingwood, ON</p><p>Phone: <a href='tel:+1705-445-3540'>+1705-445-3540</a></p></div>"],
[44.8817582, -79.3447766, "<div class='marker_info'><p>MUSKOKA OFFICE</p><p>2251 Highway 11 South, Gravenhurst, ON</p><p>Phone: <a href='tel:+1705-689-8688'>+1705-689-8688</a></p></div>"]
];
function initialize() {
var map = new google.maps.Map(document.getElementById('map'), {
zoom: 7,
center: new google.maps.LatLng(43.9982, -80.5777),
navigationControl: false,
mapTypeControl: true,
scaleControl: true
});
var bounds = new google.maps.LatLngBounds();
var infowindow = new google.maps.InfoWindow({
disableAutoPan: true
});
var customMapType = new google.maps.StyledMapType([
{
"featureType": "water",
"elementType": "geometry",
"stylers": [
{"color": "#e9e9e9"},
{"lightness": 17}
]
},
{
"featureType": "landscape",
"elementType": "geometry",
"stylers": [
{"color": "#f5f5f5"},
{"lightness": 20}
]
},
{
"featureType": "road.highway",
"elementType": "geometry.stroke",
"stylers": [
{"color": "#000"},
{"lightness": 29},
{"weight": 0.2}
]
},
{
"featureType": "road.arterial",
"elementType": "geometry",
"stylers": [
{"color": "#fff"},
{"lightness": 18}
]
},
{
"featureType": "road.local",
"elementType": "geometry",
"stylers": [
{"color": "#ffffff"},
{"lightness": 16}
]
},
{
"featureType": "poi",
"elementType": "geometry",
"stylers": [
{"color": "#f5f5f5"},
{"lightness": 21}
]
},
{
"featureType": "poi.park",
"elementType": "geometry",
"stylers": [
{"color": "#dedede"},
{"lightness": 21}
]
},
{
"elementType": "labels.text.stroke",
"stylers": [
{"visibility": "on"},
{"color": "#ffffff"},
{"lightness": 16}
]
},
{
"elementType": "labels.text.fill",
"stylers": [
{"saturation": 36},
{"color": "#333333"},
{"lightness": 40}
]
},
{
"elementType": "labels.icon",
"stylers": [
{"visibility": "on"}
]
},
{
"featureType": "transit",
"elementType": "geometry",
"stylers": [
{"color": "#f2f2f2"},
{"lightness": 19}
]
},
{
"featureType": "administrative",
"elementType": "geometry.fill",
"stylers": [
{"color": "#fefefe"},
{"lightness": 20}
]
},
{
"featureType": "administrative",
"elementType": "geometry.stroke",
"stylers": [
{"color": "#fefefe"},
{"lightness": 17},
{"weight": 1.2}
]
}
], {
name: 'Custom Style'
});
var customMapTypeId = 'custom_style';
var image = '/img/marker.png';
for (var i in LocationData) {
var p = LocationData[i];
var latlng = new google.maps.LatLng(p[0], p[1]);
bounds.extend(latlng);
var marker = new google.maps.Marker({
position: latlng,
map: map,
icon: image,
name: p[2]
});
google.maps.event.addListener(marker, 'click', function () {
infowindow.setContent(this.name);
infowindow.open(map, this);
});
}
map.mapTypes.set(customMapTypeId, customMapType);
map.setMapTypeId(customMapTypeId);
}
google.maps.event.addDomListener(window, 'load', initialize);
</script>